How to load, display and scroll external text with ActionScript 3.0.
Today we will look at how to load external text, display it and scroll it with buttons. We will work with URLRequest class, URLLoader class and use some MouseEvents. Not much to say about this topic, we will not build some fancy application, but this is very useful when working with external text.
Let’s begin.
1. Create a new file by going to File > New select Flash file (ActionScript 3.0) and click Ok.
2. Insert two new layers by going to Insert > Timeline > Layer.
3. Double click on the top layer and name it actions, name the second one buttons and the third text_field.
4. Select the text_field layer go to the Tools panel select the Text tool. On the stage click and drag to create a text field. In the Properties panel set the Text type to Dynamic the Line type to Multiline, press the show borders around text button and set the instance name to text_field. Set the other measurements like in the picture.
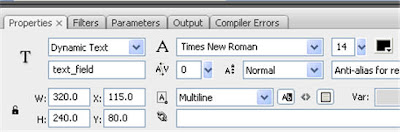
5. Select the buttons Layer. Go to Window >Common Libraries > Buttons. We will add two buttons from the Buttons Library that comes installed with Flash. Scroll down until you find the playback rounded folder. Double click on the folder to open it and select rounded green play button and drag to instance of this button on the stage.
6. Select one button go to Modify > Transform > Rotate 90 CW. Select the other button make the same steps but this time select Rotate 90 CCW. Position the buttons like in the Picture.
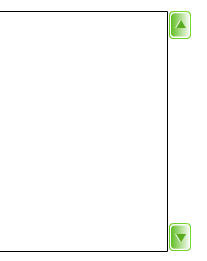
7. Select the up button set its instance name to scrollUp and for the bottom button to scrollDown.
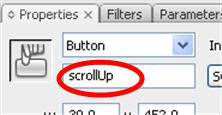
8. Save your Flash file in a new Folder and in that folder make a new txt file and paste in it some text. Paste a large amount of text because our text field it’s big and we need to have what to scroll. I named this file sample.txt.
We have finished with the visual part. Next the ActionScript code.
9.Select the first frame from the actions Layer, hit F9 to open the ActionScript panel, copy the code from bellow and paste it.
//--------------loading external text and display it---------
var textRequest:URLRequest = new URLRequest("sample.txt");
var textLoader:URLLoader = new URLLoader();
textLoader.load(textRequest);
textLoader.addEventListener(Event.COMPLETE,fileLoaded);
function fileLoaded(event:Event):void {
text_field.text = event.target.data;
text_field.wordWrap = true;
}
//------------------make buttons to scroll the loaded text-------
var scrolling:Boolean = false;
var scrollDirection:String;
scrollDown.addEventListener(MouseEvent.MOUSE_DOWN,scrollTextDown);
scrollUp.addEventListener(MouseEvent.MOUSE_DOWN,scrollTextUp);
stage.addEventListener(MouseEvent.MOUSE_UP,stopScroll);
function scrollTextDown(event:MouseEvent):void {
scrolling = true;
scrollDirection = "down";
text_field.scrollV +=1;
stage.addEventListener(Event.ENTER_FRAME,checkButtons);
}
function scrollTextUp(event:MouseEvent):void {
scrolling = true;
scrollDirection = "up";
text_field.scrollV -=1;
stage.addEventListener(Event.ENTER_FRAME,checkButtons);
}
function stopScroll(event:MouseEvent):void {
stage.removeEventListener(Event.ENTER_FRAME,checkButtons);
scrolling = false;
}
//---------ckecking to see if buttons are pressed-----------
function checkButtons(event:Event):void {
if (scrolling) {
if (scrollDirection =="down") {
text_field.scrollV +=1;
}
else if (scrollDirection == "up") {
text_field.scrollV -=1;
}
}
}
10. Hit Ctrl+Enter to test your code. It should work fain.
Let’s talk a bit about the code.
I split the code for this tutorial in three parts: loading and displaying the external text, making our buttons to scroll the text that we have loaded and checking if our buttons are hold down(if you press and not release your mouse).
In first section we create an URLRequest object and passed the path to our txt file.This object knows where our file is. Next we create an URLLoader object, this will load our txt file. We add an Event.COMPLETE to our textLoader, that will fire when our external file is loaded complete. When this happen we assign the loaded text to our text_field this process is handle by the fileLoaded function.
In the making buttons scroll text section we first declare two variables, one for keeping track is the text is scrolling (scrolling variable, this is of type Boolean, true or false) and the other for the direction in witch the text is scrolling (up or down). -After we add a MOUSE_DOWN (fires when the left button of the mouse is pressed) event to our buttons and a MOUSE_UP (fires when our left button is released) event to the stage.> -The scrollTextDown function handles (tell us what should happen when the left button of our mouse is down) the MOUSE_DOWN event added to scrollDown button. Inside this function we set our scrolling variable to true (because we are scrolling the text), set the scrollDirection variable to "down" (we are scrolling down) and increase the scrollV property of our text_field with 1.We also add an ENTER_FRAME event to the stage.
-The scrollTextUp function makes the same thing like scrollTextDown function but for the scrollUp button.
-The stopScroll function handles the MOUSE_UP event added to the stage.When this event happens we remove the ENTER_FRAME event and setting the scrolling variable to false.
In the third section, "checking if our buttons are pressed and hold down" we have the function that handles the ENTER_FRAME(fires every frame of our application) event added to the stage. Inside the checkButtons function we use a nested (one inside other)if conditional statement. First we want to know if our text is scrolling (if (scrolling), this is identical with if (scrolling ==true)) and if that is true we want to know in witch direction. If is scrolling down we add 1 to the scrollV property of the our text_field and if is scrolling up we subtract 1 from scrollV property.
And we are done with this tutorial. I hope that you enjoyed the tutorial. If you have questions, suggestions, doubts about the tutorial don’t hesitate to comment.
Until next time have fun learning Flash.
To download the source files go to:Scroll text
Comments
impressive work so hope you can help! thanks. ;o)
http://www.fuoridalcerchio.net/wordpress/tag/scrollbar/
but I there is more than one way to skin a cat ;o) Maybe you would have done something different . . . or maybe you can look at how it was made and explore it for us ... anyway thanks for your useful site.
Any ideas or tutorials to help me out with this one?
Your comments and suggestions will be trully appreciated.
Thanx a lot.
Btw, nice posts!
check this tutorial: Custom scrollbar,I think that this is what you looking for.
Java nice day
In other words, my goal is to display text001 on a mc, and when a user clicks next, I need to unload text001 and load text002 and so on...
Hope you can give me a hand (or a tip) on this one.
Thankx again.
You can’t unload the loaded text in flash. You must load a new .txt file every time you click on the next button and when it’s loaded you just assign the loaded text to your text field. This will work .
Cheers!
What code would I have to add to make the buttons disappear if the text fits within the text field?
For example, my text field is 512px high. If the text fits within that area and does not extend beyond, I want the arrows to not be there. But if I add text and make fill more area than 512 px, then I want the buttons to appear.
Is this possible?
Yes it is possible, look in the documentation at the TextField class; there is a property textHeight that holds the width of the text contained in a text field. Compare this property with the text filed height if the textHeight is bigger that text field height you will make the buttons visible.
I have updated the source files to have this capability; you can download it from here: scroll text with buttons updated