Format text with CSS in ActionScript 3.0.
Hello Flash lovers.
In the last tutorial we have learned how to load external text. In this tutorial we go a step further and we will learn how to stylize, format our text using an external CSS file. This approach offers a lot of flexibility because you can modify the CSS file at any time and your text will look different, you can do that without republishing your swf file.
Let’s see how we can accomplish that.
1. Create a new file by going to File > New select Flash file (ActionScript 3.0) and click Ok.
2. Insert a new layers by going to Insert > Timeline > Layer.
3. Double click on the top layer and name it actions, name the second one text_field.
4. Select the text_field layer, go to the Tools panel select the Text tool. On the stage click and drag to create a text field. In the Properties panel set the Text type to Dynamic, the Line type to Multiline, press the Render text as HTML button(this is important because the CSS style can be applied to Text Fields that display text as HTML) and set the instance name to tField. Set the other measurements like in the picture.
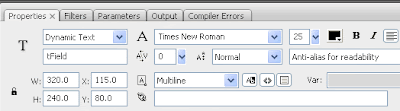
5. On your hard drive make a new folder, save the fla file in that folder. In the same folder we will put the txt file (sample.txt) and the css file (default.css).
6. Inside your new folder (where you have saved your fla file) right click >New > Text Document. Name it sample.txt. Double click to open it and paste the next text:
Txt file:
<h1>Hello everybody.</h1>
<p>Today we will look at how to load external text,display it and scroll it with buttons.We will work with <span class="boldWords">URLRequest</span> class, <span class="boldWords">URLLoader</span>class and use some <span class="boldWords">MouseEvents</span> .Not much to say about this topic, we will not build some fancy application, but this is very useful when working with external text.You can find information about how to load external text at this link:<a href="http://biochimistu.blogspot.com/2008/02/how-to-load-display-and-scroll-external.html" target="_blank">Load external text</a>.</p>
This is a simple text where I applied some HTML tags. ActionScript 3.0 doesn’t support all the HTML tags.
7. Inside the same folder make a new file, name it default.css. Double click to open it and paste next text:
I will not explain the CSS styles. For those who don’t know CSS you can find a good resource at W3 Schools.
CSS file:
h1{ font-size:24px;
font-weight:bolder;
}
p{ font-family:"Myriad Pro", "Trebuchet MS", "Lucida Sans", sans-serif;
font-size:14px;
color:#990000;
text-indent:10px;
}
.boldWords{ font-weight:bold;
font-style:italic;
}
a:link{ color:#3366FF;
text-decoration:underline;
}
a:hover{ color:#000066; }
8. In your folder you should have: the fla file, sample.txt and default.css.
9. Let’s go back to Flash. Select the first frame of the actions layer hit F9 to open the ActionScript panel copy the code from bellow and pate it.
var cssUrl:URLRequest = new URLRequest("default.css");
var cssLoader:URLLoader = new URLLoader();
cssLoader.load(cssUrl);
cssLoader.addEventListener(Event.COMPLETE,cssLoaded);
function cssLoaded(event:Event):void {
var css:StyleSheet = new StyleSheet();
css.parseCSS(URLLoader(event.target).data);
tField.styleSheet = css;
}
var textUrl:URLRequest = new URLRequest("sample.txt");
var textLoader:URLLoader = new URLLoader();
textLoader.load(textUrl);
textLoader.addEventListener(Event.COMPLETE,fileLoaded);
function fileLoaded(event:Event):void {
tField.htmlText= event.target.data;
tField.background = true;
tField.backgroundColor = 0xFFF9BF;
}
10. Hit Ctrl + Enter to test your file. It should be no problem.
Let’s look at the code.
First we create an URLRequest object this object takes as parameter the path to our file (var cssUrl:URLRequest = new URLRequest("default.css");).
On the next two lines we create an URLLoader object, (this will load our file ) and pass the path to our file (the path is hold by URLRequest object).
After we add a COMPLETE event that will fire when our file is complete loaded.
Inside the cssLoaded function that handles the COMPLETE event we create a StyleSheet object, parse the file loaded by our URLLoader as a CSS file and on the last line we setup assign the css object to the styleSheet property of the our tField.
The next code is used for loading the txt file (sample.txt) I won’t explain this again because we have looked at this code here.
This is all. I hope that you enjoyed the tutorial. If you have questions, suggestions, doubts about the tutorial don’t hesitate to comment.
Until next time have fun learning Flash.
To download the source files go to:Format text with CSS.
Comments
thanks for the tutorial.. i was searching all over for a decent tutorial and yours was the only one that works for what im doing! i too am starting out as a web designer and theres def a lot to learn. good luck with everything yo.
thanks,
allen
Where do you encounter this problem, in the source files that I provide? I have looked at the swf and it’s no problem at me. Maybe you forgot to render the text as html, this line of code:
tField.htmlText= event.target.data;
If you see the formatting, click home again and it will refresh and usually you will see the tags.
//--------------loading external text and display it---------
var cssUrl:URLRequest = new URLRequest("default.css");
var cssLoader:URLLoader = new URLLoader();
cssLoader.load(cssUrl);
cssLoader.addEventListener(Event.COMPLETE,cssLoade d);
function cssLoaded(event:Event):void {
var css:StyleSheet = new StyleSheet();
css.parseCSS(URLLoader(event.target).data);
text_field.styleSheet = css;
}
var textRequest:URLRequest = new URLRequest("home.txt");
var textLoader:URLLoader = new URLLoader();
textLoader.load(textRequest);
textLoader.addEventListener(Event.COMPLETE,fileLoa ded);
function fileLoaded(event:Event):void {
text_field.text = event.target.data;
text_field.wordWrap = true;
}
any ideas? Dave
wondering if you might show what the code would look like for a Flash file containing 2 different html text boxes -
mutiple text boxes, styled by a single CSS file, really starts to demo the power of CSS.
I'm not sure what I need to copy/paste and modify
many mahalos
this is not complicated; fallow the step 4 from the tutorial to create a new text field, set its instance name to tField2.Inside the folder where its sample.txt file make a new txt file, name it sample2.txt paste some text and apply the desired html tags. Open the css file and add the formatting for the sample2.txt.
At the existing code add:
var textUrl2:URLRequest = new URLRequest("sample2.txt");
var textLoader2:URLLoader = new URLLoader();
textLoader2.load(textUrl2);
textLoader2.addEventListener(Event.COMPLETE,fileLoaded);
and inside the fileLoaded function place:
tField2.htmlText= textLoader2.data;
I didn’t test the code, but it should work.
Sorry for the delay.
I've noticed on slower internet connections it takes a couple of moments for the text to render on the screen - have you observed this before? Would you know how to speed things up (or add a preloader?)
Thanks,
Kartik
Yes it could take some time to load the text when you have large chunks of text. You can add a preloader if you load large text files, you can find on this blog a tutorial about preloaders.
It works perfectly when I publish the swf b itself. However if I load the swf with the ccs formatted text in it into a parent, the text is not visible.
Any ideas as to why this is? I'd really appreciate any help here, this method of loading text is by far the simplest and user friendly I've come across so I'd like to keep it.
Many thanks, Vitamin D
what does it say, you get some kind of error or just the text it's not showing?